SSL for Astyanax
Security is important for any application that handles personal data and one of the most common ways of protecting the wire is through the use of SSL. Because of my struggle to setup client- server SSL I though I'd share what I learned in the form of a brief tutorial on the steps necessary to implement client-server security between Cassandra and the Astyanax client.
Firstly to clarify the terms used.
Astyanax - A high-level, thrift based Java API for Cassandra.
Java Keystore (JKS) - A file that stores private keys, and the certificates with their corresponding public keys.
Java Truststore (JTS) - Another file that contains certificates from other parties that you expect to communicate with, or from Certificate Authorities that you trust to identify other parties.
Keytool - A key and certificate management utility allowing for creation and signing of certificate stores.
Secure Sockets Layer (SSL) - cryptographic protocol that provides communication security over the Internet.
Creating the Necessary Certificates
The simplest guide out there was Acunu's guide to Cassandra security. The section headed 'Cassandra client certificates' contains detailed information and explanation on the various keytool commands used to generate the necessary certificate stores and are the source of the below snippet of code outlining the process:
Configuring Cassandra to use SSL
The configuration takes place in the cassandra.yaml file located in casandradir/conf/ We are after the following option client_encryption_options (typically located near the bottom of the file) which should be modified to the below snippet:
Enabling SSL in the Astyanax client
To allow Astyanax to communicate with Cassandra the password and path to the certificate store has to be supplied when creating the Astyanax context.
The file containing the certificate store, based on the above certificate generation example, is "cassandra_external_trust.jks".
Within the creation of the Astyanax context, in the withConnectionPoolConfiguration method we call setSSLConnectionContext to enable SSL and pass a SSLConnectionContext object as a parameter. The object requires two parameters, the part to the certificate store and the store’s password:
And now our communication channel is protected by encryption via SSL. The below two screen shots of Wireshark will hopefully demonstrate why it's a good idea to protect your communication channel.
Regular Thrift traffic
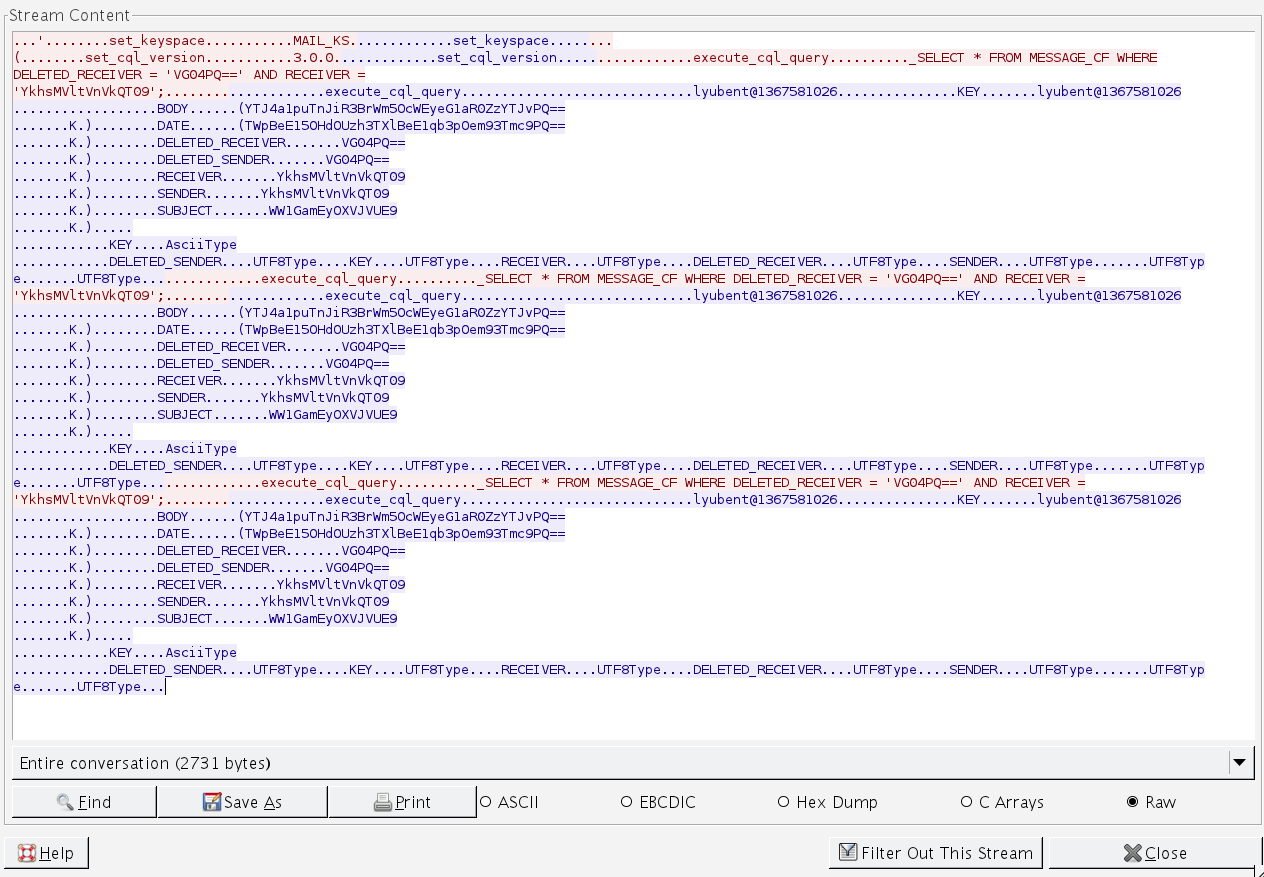
Encrypted Thrift traffic
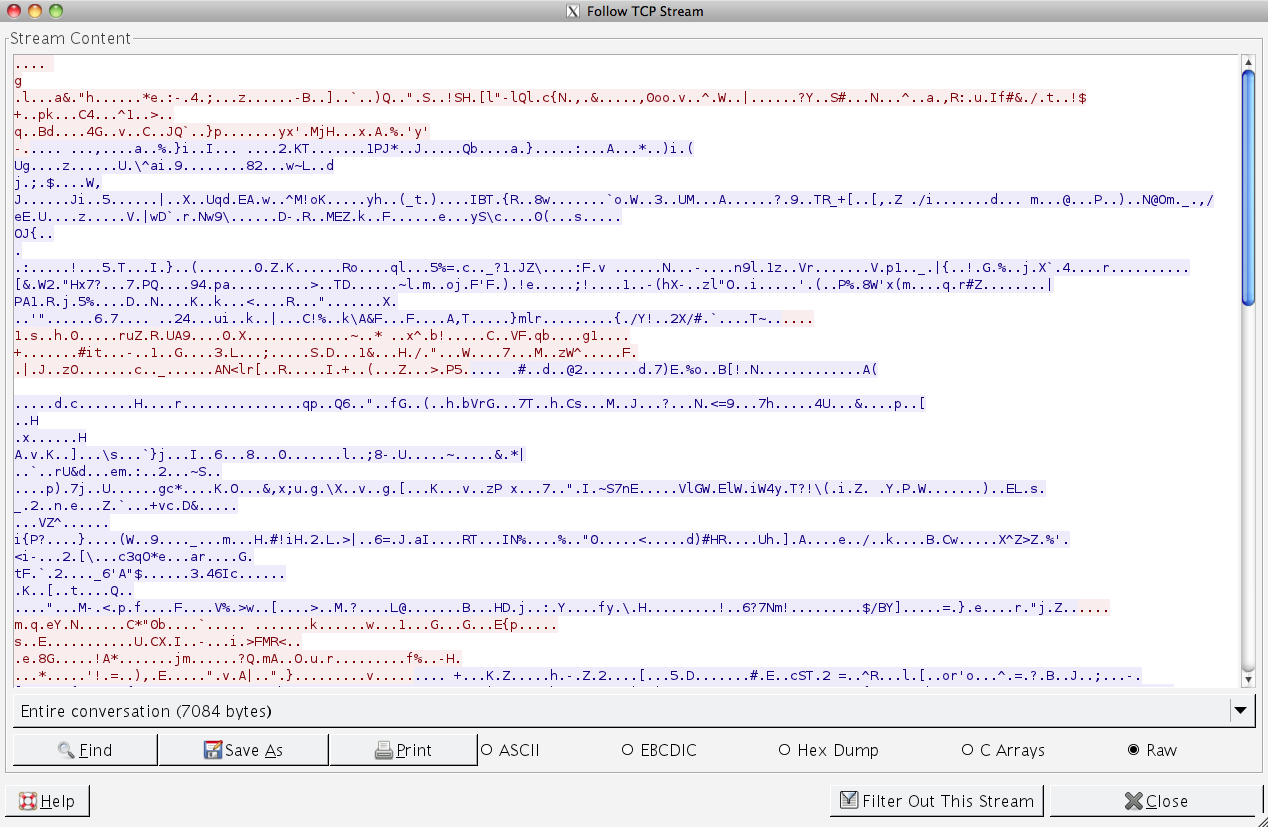